Loading Please Wait ....
L.C.W.D
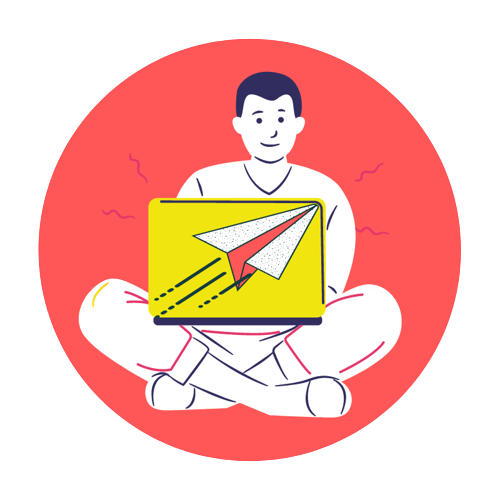
Learn Code With Durgesh
Learn Code With Durgesh offers wide variety of free and Premium courses on YouTube channel and website. We are serving lakhs of students and professionals.
!! Happy Coding !!
Products
Contact
Substring Technologies, Vijayeepur ,
Vishesh Khand 2 Gomti Nagar, Lucknow, INDIA, 226010
+91-9839466732
Copyright © 2023: Substring Technologies Pvt Ltd.
All Rights Reserved.